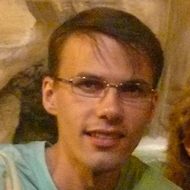
Liferay upload files programmatically in java
by Stanislav on Friday Jul 17, 2015
If you have a requirement to implement a file upload form in your Liferay portlet then this article for you. In this post I will provide a simple upload jsp form and java code snippet that handles file upload action and stores uploaded file to Liferay document library.
Upload form written in JSP looks like:
<portlet:actionURL var="portletActionURL" /> <aui:form action="<%= portletActionURL %>" method="POST" enctype="multipart/form-data"> <aui:row> <aui:column columnWidth="50"> <input type="file" class="multi" maxlength="10" name="attachedFile"/> </aui:column> </aui:row> <aui:button-row> <aui:button type="submit" /> </aui:button-row> </aui:form>
What should be mentioned here:
- portletActionURL – is a url generated by portlet:actionURL tag. This url points to the portlet action handler that controls all action requests. In our case javax.portlet.ActionRequest will be analysed whether there is file attached or not. And if file is found when it will be stored to Liferay document library.
- method=”POST” – we always should specify POST method (not GET or other method) when we deal with file upload.
- enctype="multipart/form-data" and <input type="file"> - if we want to develop a client side file upload and <input type=”file”> tag given, when we should always encode the content of the request with multipart/form-data.
- for every upload form, a submit button should exist.
- input file field has a class “multi”. It took me some time to find a good javascript plugin that provides multiple file upload on submit action (with the ability to remove added files). Finally I’ve found jquery-multifile tiny library that has simple but sufficient graphical user interface. To plug this library to input file field the class=“multi” should be added.
Java portlet action handler that receives client requests on submit will have the following methods - first we need to check if the file exists in incoming request:
public static boolean isAttachExistsInRequest(final UploadPortletRequest req) { final FileItem[] arr = req.getMultipartParameterMap().get( "attachedFile"); if (arr == null || arr.length == 0) { return false; } boolean isAttachExists = false; for (final FileItem fi : arr) { // fileName == "" when no file input (type=file) has no files // uploaded if (!fi.getFileName().isEmpty()) { isAttachExists = true; } } return isAttachExists; }
Second, if we’ve found that the files exist in request when we need to store them:
public static void storeAttachments(final UploadPortletRequest req) throws PrincipalException, Exception { if (isAttachExistsInRequest(req)) { final FileItem[] arr = req.getMultipartParameterMap().get( "attachedFile"); final long repoId = getRepositoryId(req); final long folderId = getSomeFolder(); final ServiceContext sc = new ServiceContext(); for (final FileItem fi : arr) { createFileEntry(repoId, folderId, fi.getFileName(), fi.getContentType(), fi.getSize(), fi.getInputStream(), sc); } } } private static long getRepositoryId(final UploadPortletRequest req) { final ThemeDisplay themeDisplay = (ThemeDisplay) req .getAttribute(WebKeys.THEME_DISPLAY); return themeDisplay.getScopeGroupId(); } public static void createFileEntry(final long repositoryId, final long folderId, final String fileName, final String mimeType, final long fileLength, final InputStream is, final ServiceContext serviceContext ) { try { final FileEntry entry = DLAppServiceUtil.addFileEntry(repositoryId, folderId, fileName, mimeType, fileName, null, null, is, fileLength, serviceContext); } catch (final Exception e) { log.error("Utils::createFileEntry Exception", e); } }
And in the end here is the final look of the file uploader:
Tags: liferay
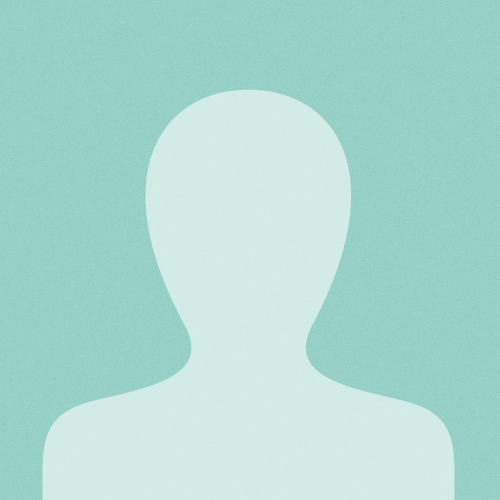
Stan Aug 17, 2015 at 13:42 #
Hassan, you should implement org.springframework.web.portlet.mvc.Controller. And put mentioned methods to handleActionRequest(ActionRequest request, ActionResponse response). Detailed info here: http://soft29.info/blog/entry/liferay-spring-mvc-portlet-jpa
Hassan Aug 10, 2015 at 19:11 #
could you post the source code does this methods you write are callback functions or how i could youse them ??? thanks